Share
Tweet
Share
Share
In the ever-evolving landscape of web development, creating reusable components is pivotal for building scalable and maintainable applications. React, with its component-based architecture, has been a game-changer in this realm.
However, integrating powerful tools like ReExt can further enhance your development workflow, making the creation of custom reusable components even more efficient and robust. This guide will walk you through building reusable components in React using ReExt, catering to both newcomers and seasoned developers
Introduction to ReExt
ReExt is a powerful extension for React developers provided by Sencha, designed to streamline the process of building reusable components. By leveraging ReExt, developers can enhance their productivity, maintain consistent code standards, and create components that are easy to manage and scale. Whether you’re a newcomer to React or a seasoned developer, ReExt offers tools and configurations that can significantly improve your development workflow.
Setting Up Your Development Environment
Before diving into ReExt, it’s essential to ensure that your development environment is correctly set up. This involves installing necessary software like Node.js and npm (Node Package Manager), which are fundamental for managing dependencies and running your React applications.
Installing Node.js and npm
1. Download Node.js: Visit the official Node.js website and download the LTS (Long Term Support) version suitable for your operating system.
2. Install Node.js: Run the downloaded installer and follow the on-screen instructions. This process will also install npm automatically.
3. Verify Installation: Open your terminal or command prompt and run the following commands to verify the installation:
node -v
npm -v
You should see the versions of Node.js and npm displayed.
Installing ReExt
ReExt can be installed via npm, the package manager for JavaScript. The installation process varies slightly depending on whether you’re a TRIAL or ACTIVE customer.
If You Are a TRIAL Customer
For those evaluating ReExt, the 30-day trial package is available publicly on npm. To install the latest version of ReExt, run:
npm install @sencha/reext
If You Are an ACTIVE Customer
Active customers have access to Sencha’s private npm registry. To install ReExt:
1. Login to Sencha’s Registry:
Replace name..gmail.com with your actual username, where the @ symbol is replaced by two periods.
npm login –registry=https://npm.sencha.com/ –scope=@sencha
2. Install ReExt:
npm install @sencha/reext
Note: Ensure that your username is correctly formatted, replacing the @ symbol with two periods as specified in the installation instructions.
Using the ReExt Designer Extension in Visual Studio Code
To maximize the benefits of ReExt, it’s recommended to use the ReExt Designer Extension within Visual Studio Code (VS Code). This extension provides a visual interface for designing and managing your components, making the development process more intuitive.
Installing the Extension
1. Download the Extension: Obtain the preview version of the ReExt Designer Extension from the provided source.
2. Install in VS Code:
○ Open VS Code.
○ Navigate to the Extensions Activity Bar.
○ Click the ‘…’ menu in the upper right corner of the Extensions View.
○ Select ‘Install from VSIX…’ and choose the downloaded extension file.
3. Activate the Extension: Once installed, the extension should activate automatically, providing additional tools and menus within VS Code tailored for ReExt.
Quick Start with ReExt
ReExt supports various React setups, including Vite, Next.js, and Create React App. Below are step-by-step instructions to get started with each setup.
Vite
Vite is a fast and modern build tool for front-end development. To set up a ReExt project with Vite:
Create a New Vite Projectnpm create vite@latest reextvite — –template react-swcNavigate to Project Directory:
cd reextvite
Install ReExt:
npm install @sencha/reext@latest
Copy Configuration and Example Files
cp node_modules/@sencha/reext/dist/example/vite.config.js vite.config.js
cp node_modules/@sencha/reext/dist/example/App.jsx src/App.jsx
cp node_modules/@sencha/reext/dist/example/main.jsx src/main.jsx
Run the Project:
npx vite –open
Next.js with JavaScript
Next.js is a popular React framework for building server-side rendered applications.
1. Create a New Next.js Project:
npx create-next-app@latest reextnext –js –eslint –no-tailwind –no-src-dir –app –import-alias “@/*”
Navigate to Project Directory
cd reextnext
Install ReExt:
npm install @sencha/reext@latest
Copy Configuration and Example Files
cp node_modules/@sencha/reext/dist/example/next.config.mjs next.config.mjs
cp node_modules/@sencha/reext/dist/example/layout.js app/layout.js
cp node_modules/@sencha/reext/dist/example/page.js app/page.js
Run the Project
npm run dev
Create React App
Create React App is a widely-used tool for setting up React projects quickly.
1. Create a New Create React App Project:
npx create-react-app reextcra
2. Navigate to Project Directory:
cd reextcra
3. Install ReExt:
npm install @sencha/reext@latest
4. Copy Configuration and Example Files:
cp node_modules/@sencha/reext/dist/example/vite.config.js vite.config.js
cp node_modules/@sencha/reext/dist/example/App.jsx src/App.js
cp node_modules/@sencha/reext/dist/example/main.jsx src/index.js
5. Run the Project:
6. npm start
Building Reusable Components with ReExt
Creating reusable components is an art that combines good design principles with efficient tooling. ReExt augments React’s capabilities, providing additional configurations and structures that simplify component creation.
Understanding Reusable Components
Reusable components are modular pieces of UI that can be used across different parts of an application without modification. They promote consistency, reduce code duplication, and enhance maintainability.
Creating a Component
With ReExt installed, creating a new component involves leveraging ReExt’s configuration options to define the component’s behavior, appearance, and integration with the rest of the application.
1. Define the Component Structure: Decide on the component’s purpose and structure. For example, a button, a form input, or a modal dialog.
2. Create Configuration Options: Use ReExt’s config member type to define configurable properties for the component.
3. Implement Methods and Events: Utilize ReExt’s method and event member types to define the component’s behavior and interactions.
4. Style with Theme Variables: Incorporate ReExt’s theme variable member types to ensure consistent styling across components.
5. Manage Access Levels: Use ReExt’s access level descriptors to control the visibility and extensibility of component members.
Adding Configuration Options
Configuration options allow components to be flexible and adaptable to different contexts. ReExt facilitates defining these options through its config member type.
● Config Definition:
config: {
text: {
type: String,
default: ‘Click Me’,
required: true
},
onClick: {
type: Function,
default: () => {}
}
}
● Usage: These configs can be passed as props when using the component, allowing for customization.
Using Getter and Setter Methods
Getter and setter methods allow controlled access to component properties. ReExt automatically generates these methods based on the defined config options.
● Getter Example:
getText() {
return this.text;
}
● Setter Example:
setText(newText) {
this.text = newText;
return this;
}
● Benefits: These methods provide a standardized way to access and modify component properties, enhancing encapsulation and maintainability.
Styling with Theme Variables
Consistent styling is crucial for maintaining a cohesive look and feel across an application. ReExt’s theme variables enable centralized management of styling properties.
● Defining Theme Variables:
jsx
Copy
themeVariables: {
primaryColor: ‘#007bff’,
secondaryColor: ‘#6c757d’
}
● Applying Theme Variables:
const styles = {
button: {
backgroundColor: this.primaryColor,
color: ‘#fff’,
border: ‘none’,
padding: ’10px 20px’,
cursor: ‘pointer’
}
};
● Advantages: Theme variables ensure that changes to design are propagated consistently across all components that use them.
Managing Access Levels
Access levels define the visibility and accessibility of component members (methods, properties). ReExt supports three access levels: public, protected, and private.
● Public: Members are accessible from any other part of the application.
● Protected: Members are accessible only within the component and its subclasses.
● Private: Members are for internal use within the component and are not accessible externally.
● Example:
class MyComponent extends ReExt.Component {
// Public method
public doSomething() {
// …
}
// Protected method
protected helperMethod() {
// …
}
// Private method
private computeValue() {
// …
}
}
● Best Practices: Properly managing access levels enhances encapsulation, reduces the risk of unintended interactions, and makes the component’s API clearer.
Example: Building a Custom Button Component
To illustrate the concepts discussed, let’s build a custom button component using React and ReExt. This component will be reusable, configurable, and styled consistently using ReExt’s theme variables.
Step 1: Setting Up the Project
Assuming you have already set up a React project with ReExt installed (refer to the Quick Start with ReExt section), proceed to create your custom component.
Step 2: Creating the Button Component
1. Create a New File: In your src directory, create a new file named CustomButton.jsx.
2. Import Necessary Modules:
import React from ‘react’;
import ReExt from ‘@sencha/reext’;
3. Define the Component:
class CustomButton extends ReExt.Component {
constructor(props) {
super(props);
this.config = {
text: ‘Click Me’,
onClick: () => {}
};
}
render() {
const styles = {
button: {
backgroundColor: this.themeVariables.primaryColor,
color: ‘#fff’,
border: ‘none’,
padding: ’10px 20px’,
cursor: ‘pointer’,
borderRadius: ‘4px’,
fontSize: ’16px’
}
};
return (
<button style={styles.button} onClick={this.onClick}>
{this.text}
</button>
);
}
}
export default CustomButton;
Step 3: Adding Configuration and Props
Enhance the CustomButton component by defining configuration options and utilizing getter and setter methods.
1. Define Configurations:
class CustomButton extends ReExt.Component {
constructor(props) {
super(props);
this.config = {
text: {
type: String,
default: ‘Click Me’,
required: true
},
onClick: {
type: Function,
default: () => {}
}
};
}
// Getter
getText() {
return this.text;
}
// Setter
setText(newText) {
this.text = newText;
return this;
}
// Getter for onClick
getOnClick() {
return this.onClick;
}
// Setter for onClick
setOnClick(newOnClick) {
this.onClick = newOnClick;
return this;
}
render() {
// … (same as before)
}
}
2. Usage in Parent Component:
import React from ‘react’;
import CustomButton from ‘./CustomButton’;
const App = () => {
const handleClick = () => {
alert(‘Button Clicked!’);
};
return (
<div>
<CustomButton text=”Submit” onClick={handleClick} />
<CustomButton text=”Cancel” onClick={() => console.log(‘Cancel Clicked’)} />
</div>
);
};
export default App;
Step 4: Styling the Button
Use ReExt’s theme variables to manage the styling of the CustomButton component.
1. Define Theme Variables:
class CustomButton extends ReExt.Component {
constructor(props) {
super(props);
this.config = {
// … (same as before)
};
this.themeVariables = {
primaryColor: ‘#007bff’
};
}
// … (same as before)
}
2. Apply Theme Variables in Styles:
render() {
const styles = {
button: {
backgroundColor: this.themeVariables.primaryColor,
// … (same as before)
}
};
// … (same as before)
}
3. Updating Theme Variables: To change the button’s primary color across all instances, simply update the primaryColor in the theme variables.
Step 5: Using the Button in Your App
With the CustomButton component defined, integrating it into your application is straightforward.
1. Import and Use the Component:
import React from ‘react’;
import CustomButton from ‘./CustomButton’;
const App = () => {
const handleSubmit = () => {
console.log(‘Submit Button Clicked’);
};
const handleCancel = () => {
console.log(‘Cancel Button Clicked’);
};
return (
<div style={{ padding: ’20px’ }}>
<h1>ReExt Custom Button Example</h1>
<CustomButton text=”Submit” onClick={handleSubmit} />
<CustomButton text=”Cancel” onClick={handleCancel} />
</div>
);
};
export default App;
2. Run the Application:
npm start
Your application should now display two buttons—”Submit” and “Cancel”—each responding to clicks as defined.
Tips for Newbies and Experienced Developers
For Newbies
● Understand the Basics: Before diving into ReExt, ensure you have a solid understanding of React’s fundamentals, including components, props, and state.
● Leverage Documentation: Utilize the ReExt Getting Started Guide to familiarize yourself with ReExt’s features and configurations.
● Start Simple: Begin by creating simple components and gradually incorporate more advanced features like configuration options and theme variables.
For Experienced Developers
● Optimize Reusability: Design components with reusability in mind, ensuring they are flexible and configurable for various use cases.
● Utilize Advanced Configurations: Take advantage of ReExt’s advanced configurations, such as access levels and member flags, to create robust and maintainable components.
● Integrate with Existing Workflows: Seamlessly integrate ReExt into your existing development workflows, leveraging tools like the ReExt Designer Extension for enhanced productivity.
Best Practices
1. Consistent Naming Conventions: Use clear and consistent naming for components, props, and methods to enhance readability and maintainability.
2. Encapsulation: Encapsulate component logic and styling to prevent unintended side effects and promote modularity.
3. Documentation: Document your components thoroughly, describing their configurations, methods, and usage examples.
4. Theming: Utilize theme variables for styling to ensure a consistent look and feel across your application and facilitate easy theme changes.
5. Access Control: Properly manage access levels for component members to maintain a clean and secure API.
Conclusion
Building reusable React UI components is a cornerstone of efficient and scalable React applications. By integrating ReExt into your development workflow, you can elevate your component design, ensuring they are not only reusable but also configurable, maintainable, and consistent.
Whether you’re just starting with React or you’re an experienced developer looking to refine your component architecture, ReExt offers the tools and configurations necessary to enhance your development process. Embrace ReExt today and take your React UI components to the next level!
Bring Ext JS to React Seamlessly – Experience ReExt for Free!
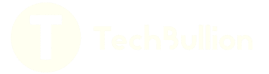